Decision Structure
Decisions in a program are used when the program has conditional choices to execute a block of code.
Decision structures evaluate multiple expressions and return True or False as the result. You need to determine
which action to take and which statements to execute if the result is True or False.
The Python Boolean type has only two possible values: True and False.
We are going to work on three types of decision making structures.
1. If statements
2. If-else statements
3. Nested if-else statements
When working with decision conditions, we need relational operators.
A relational operator checks whether its operands satisfy a specified relationship and produces a Boolean value based on its assessment.
It returns True or False.
For example, the value of the expression 3 < 9 is True because the < operator is the "less than" operator.
The expression x < y has the value True if the value of x is smaller than the value of y and False otherwise.
The following table lists several commonly used Boolean operators in Python.
Let's look at some examples of Comparison Operators.
x = 24
y = 69
print('x > y is', x>y)
# Output: x > y is False
print('x < y is', x<y)
# Output: x < y is True
print('x >= y is',x>=y)
# Output: x >= y is False
print('x <= y is',x<=y)
# Output: x <= y is True
print('x == y is',x==y)
# Output: x == y is False
print('x != y is',x!=y)
# Output: x != y is True
Check the following links for more relational operators and images.
https://creativecomputing.ca/11/11_1_1_Relational_Operators.html
https://towardsdatascience.com/python-operators-from-scratch-a-beginners-guide-8471306f4278
if, if else, nested if
if
if statement is the most simple decision making statement. If the condition is True, then a block of statements is executed.
If the condition is False, the block of statements is not executed.
The following example has one if condition, and it evaluates when the disc value is greater than and equal to zero.
It does nothing when the disc value is less than 0.
#Solve the quadratic equation ax**2 + bx + c = 0
import numpy as np
def main():
a,b,c = eval(input("Enter coefficient a,b,c, separated by commas: "))
# calculate the discriminant
disc = (b**2) - (4*a*c)
if disc >= 0:
#find two solutions
root1 = (-b-np.sqrt(disc))/(2*a)
root2 = (-b+np.sqrt(disc))/(2*a)
print("The two roots of the quadratic equation are {0:0.2f} and {1:0.2f}".format(root1,root2))
main()
Output:
Enter coefficient a,b,c, seperate by comma: 1,5,6
The two roots of the quadratic equation are -3.0 and -2.0
if else
The block of code is executed when the condition is True. If the condition is False, another block of code is executed.
# Solve the quadratic equation ax**2 + bx + c = 0
# import complex math module
import numpy as np
def main():
a,b,c=eval(input("Enter coefficient a,b,c, separated by commas: "))
# calculate the discriminant
disc = (b**2) - (4*a*c)
if disc < 0:
print("The equation has no real roots.")
else:
# find two solutions
root1 = (-b-np.sqrt(disc))/(2*a)
root2 = (-b+np.sqrt(disc))/(2*a)
print("The two roots of the quadratic equation are {0:0.2f} and {1:0.2f}".format(root1,root2))
main()
Output no real roots if the disc value is less than zero.
Enter coefficient a,b,c, separate by comma: 1,2,3
The equation has no real roots.
-------------------------------------------------
Enter coefficient a,b,c, separate by comma: 2,16,4
The two roots of the quadratic equation are -7.74 and -0.26
if, elif, else
The elif is short for else if. It allows us to check for multiple expressions.
If the condition for if is False, it checks the condition of the next elif block and so on.
If all the conditions are False, the body of else is executed. Only one block among the several if...elif...else blocks is executed according to the condition.
Note:The if block can have only one else block, however it can have multiple elif blocks.
# Solve the quadratic equation ax**2 + bx + c = 0
# import complex math module
import numpy as np
def main():
a,b,c = eval(input("Enter coefficient a,b,c, separate by comma: "))
# calculate the discriminant
disc = (b**2) - (4*a*c)
if disc < 0:
print("The equation has no real roots.")
elif disc == 0:
root = -b/(2*a)
print("There is a double root at", root)
else:
root1 = (-b-np.sqrt(disc))/(2*a)
root2 = (-b+np.sqrt(disc))/(2*a)
print("The two roots of the quadratic equation are {0:0.2f} and {1:0.2f}".format(root1,root2))
main()
Sample output is listed here
Enter coefficient a,b,c, separate by comma: 1,2,3
The equation has no real roots.
Enter coefficient a,b,c, separate by comma: 1,2,1
There is a double root at -1.0
Enter coefficient a,b,c, separate by comma: 1,8,2
The two roots of the quadratic equation are -7.74 and -0.26
Images are done by Gaurav Tiwari
https://www.pyforschool.com/tutorial/decision-structure.html
Boolean Operators
Python provides three Boolean operators: and, or and not.
The Boolean operators and and or are used to combine two Boolean expressions and produce a Boolean result: True or False.
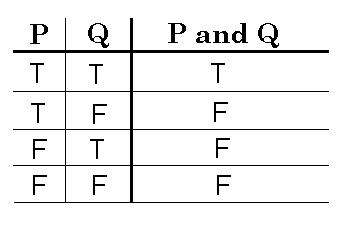
https://www.fallacies.ca/ttable.htm
https://cs.stanford.edu/people/nick/py/python-boolean.html
Logical Operators
x = True
y = False
print('x and y is',x and y)
#output: x and y is False
print('x or y is',x or y)
#Output: x or y is True
print('not y is',not y)
#Output: not y is True
print('not x is',not x)
#Output: not x is False
The following example asks the user to enter three numbers and outputs the largest number.
def main():
x,y,z= eval(input("Enter three numbers separated by commas: "))
if x > y and x > z:
largest = x
elif y > x and y > z:
largest = y
else:
largest = z
print("The largest number is",largest)
main()
Output
Enter three numbers separate by comma: 56,-12,197
The largest number is 197
https://www.w3schools.com/python/python_operators.asp
Lab Assignment
1. Write a program to compute the total wages for the week. The requirement is you will receive pay time-and-a-half for
any hours worked over 40 hours in a given week.
Requirement:
- Input hours worked and hourly rate.
- Calculate number of hours overworked and the extra pay for working over 40 hours per week
- Calculate total number of pay per week.
Sample output. The output values are required to be set in 2 decimal places.
Welcome to our weekly pay calculator
Enter hours worked: 25
Enter hourly wage: 12
Your week's total pay is $300.00
---------------------------------------------------
Welcome to our weekly pay calculator
Enter hours worked: 57
Enter hourly wage: 12
You worked extra 17.0 hours per week. You are getting $306.00 for the extra hours.
Your week's total pay is $786.00
2. Fix and complete the following temperature conversion program.
Requirement:
- Make sure both letter 'c' or 'C' are valid input to convert from Celsius to Fahrenheit.
- Make sure both letter 'f' or 'F' are valid input for Fahrenheit to Celsius.
- If the input letter is not c,C,f, or F, output "Invalid letter, please enter c,C,f, or F".
def main():
print("Input Menu:")
print("C: Celsius to Fahrenheit.")
print("F: Fahrenheit to Celsius.")
letter = input("letter :")
if letter == 'c':
c=float(input("Temperature in Celsius: "))
f=1.8*(c)+32.0
f=round(f,2) #another way to round to 2 decimal place
print("Temperature in Fahrenheit:",f)
elif letter == 'f'
f=float(input("Temperature in Fahrenheit: "))
c=(f-32)/1.8
c=round(c,2)
print("Temperature in Celsius:",c)
main()
Sample output. The output values are required to be set in 2 decimal places.
Input Menu:
C: Celsius to Fahrenheit.
F: Fahrenheit to Celsius.
letter : c
Temperature in Celsius: 0
Temperature in Fahrenheit: 32.0
---------------------------------
Input Menu:
C: Celsius to Fahrenheit.
F: Fahrenheit to Celsius.
letter : F
Temperature in Fahrenheit: 70
Temperature in Celsius: 21.11
--------------------------------
.
Input Menu:
C: Celsius to Fahrenheit.
F: Fahrenheit to Celsius.
letter : r
Invalid letter, please enter either c or f.